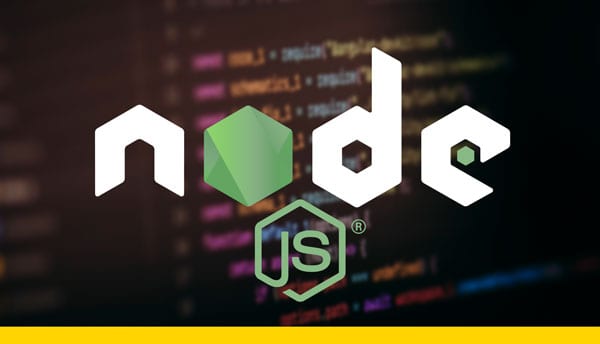
x
Course: Outline
Course Introduction
- Overview of Node and the course
- Connecting to class virtual machines
- Student and Instructor Introductions
- Node Development Environment Setup
- Basics of Git
- Overview of Visual Studio Code (VS Code)
- How markdown files are used in projects
- Committing code in your own branch
Executing Scripts with Node
- Installing and updating Node
- Ways to execute JavaScript using Node
- ES6 features: including ES6 classes
- Practicing with Array.prototype functions
- Review of arrow functions
Modules, globals & event loop
- What are modules
- Using the CommonJS interface for modules
- How to require custom modules
- Using the event loop
- How to require core modules
- Understanding the Event Loop
- Using the Event Emitter
Using the Node Package Manager (npm)
- Background of NPM and its usage
- The meaning and usage of packages
- Understanding how to use semver
- Creating and using package.json files
- Installing, Listing, Uninstalling and Updating Modules
- Global vs. local package installation
- When to use --save and --save-dev
Working with the filesystem and Promises
- Require-ing core file modules
- Relative path information
- Using the fs module to:
- open, read, write files
View and create directories
- Working with Promises
- Working with Streams
- Writing and Reading from Stream Resources
- Piping and Chaining Streams
- Using buffers for binary data
Database Access with Node
- Working with the PostgreSQL database
- Overview of course database
- Using Knex as a Query Builder
- Creating a database and tables with Node
- Database CRUD with Node
- Creating a Migration for managing database changes
Creating a Simple HTTP Server to receive requests
- Reviewing the HTTP protocol
- Discussing HTTP Request methods
- Reviewing Response Codes
- Creating a HTTP basic server
- Installing and using nodemon for faster development
Getting Started with the Express Module
- Overview of Express middleware framework
- Using Express to create a server
- Creating Middleware
- Creating routes for GET, POST, PUT, PATCH,DELETE
- Creating a 404 route
- Creating a Rest API
Audience
Experienced JavaScript developers who wish to understand Node JS
Prerequisites
- Programming experience with JavaScript is required.
- Knowledge of REST based web services is helpful but not required.
What You Will Learn
After successfully completing this course, students will be able to:
- Understand Node.js fundamentals & features.
- Write custom CommonJS and ES6 Modules.
- Install and setup Node projects using npm.
- Use Node to work with files and databases.
- Leverage common core modules of Node.
- Create Express servers and work with middleware.
- Manage module dependencies / packageswith package.json files.
- Build a Rest API using Node, Express and Postgres.