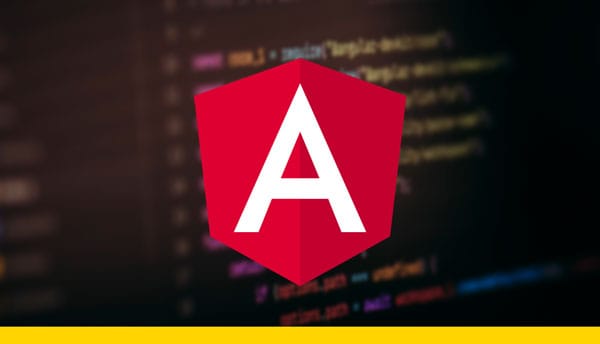
Overview of Course and Angular Development
- An Overview of Angular
- Course Logistics
- Using Git to get the course labs and demos
- Basics of VS Code
- Creating a branch for your Angular work
Creating and Exploring Angular Projects
- ES6 Syntax, Modules and TypeScript
- Using Angular CLI to create new projects
- Overview of Angular project files
- Use of decorators, root module and component
- Structural Files to build Angular projects
- How Angular starts up
- Modifying starter projects
- Adding Bootstrap as a project dependency
- Changing the default port and favicon
Data Binding in Components
- Creating more components
- What makes up a Component decorator
- Type Checking Data
- Data Binding using {{interpolation}} and [property binding]
- Using template expressions
- Debugging Angular Apps with Angular Augury
- A Overview of Change Detection
Passing Data & Event Binding
- Reviewing properties of @NgModule
- Passing data from Parent to Child with @Input()
- Binding Events
- Using @Output() to call from child to parent
Using Directives
- Using Built-in Directives
- Using Structural Directives: *ngFor *nglf *ngSwitch
- Optimizing rendering of data
- Bonus: Adding Style to Components
- Bonus: Displaying code with ngNonBindable
Using and Creating Pipes
- Using built-in pipes to transform data
- currency, decimal, date, uppercase, lowercase
- Passing parameters to pipes
- Debugging with JSON pipe
- Creating custom pipes
Understanding Change Detection and Lifecycle hooks
- Changing Detection strategies: Default and OnPush
- Demonstration of lifecycle hooks being called
- Preventing memory leaks with OnDestroy
Services, HttpClient and Observables
- Creating services
- How to register services with providers
- Dependency injection via constructors
- How to make API calls using HttpClient
- Working with Observables and RxJS
Leveraging Routing Features
- Setup Basic Routing and feature modules
- How to navigate between routes
- How to style active routes using a directive
- How to configure Parameterized Routes in our route definition object
- Use Lazy Loading
- Configuring Child Routes
- Using Route Guards to prevent navigation to/from components
Working with Forms
- Comparing differences between template-driven and reactive forms
- Creating two-way data bindings with ngModel
- Creating and using template reference variables
- Handling form validation with Angular
Unit Testing in Angular
- Creating a unit test suite with Karma, Jasmine, and TypeScript
- Writing isolated unit tests for pipes and services
- Configuring TestBed
Students who wish to gain a fundamental understanding of Angular components and modules and wish to create their own Angular applications from a set of requirements.
This is a hands-on programming class. Attendees must have previous working
experience with web development.
Equivalent knowledge which includes working with VS Code extensions and shortcuts, HTML5 form elements and attributes, JavaScript / EcmaScript 6 (let / const, backticks, spread operators, ES6 modules).
CSS selectors is helpful, but not required for training.
- Use TypeScript, ES6 notations and Angular CLI to create apps
- Create components and use data binding, pipes & directives in templates
- Utilize event binding and component communications
- Create Services and use HttpClient to make AJAX calls
- Work with the Angular Router
- Differentiate between Reactive and Template Driven Forms
- Introduce Unit Testing in Angular